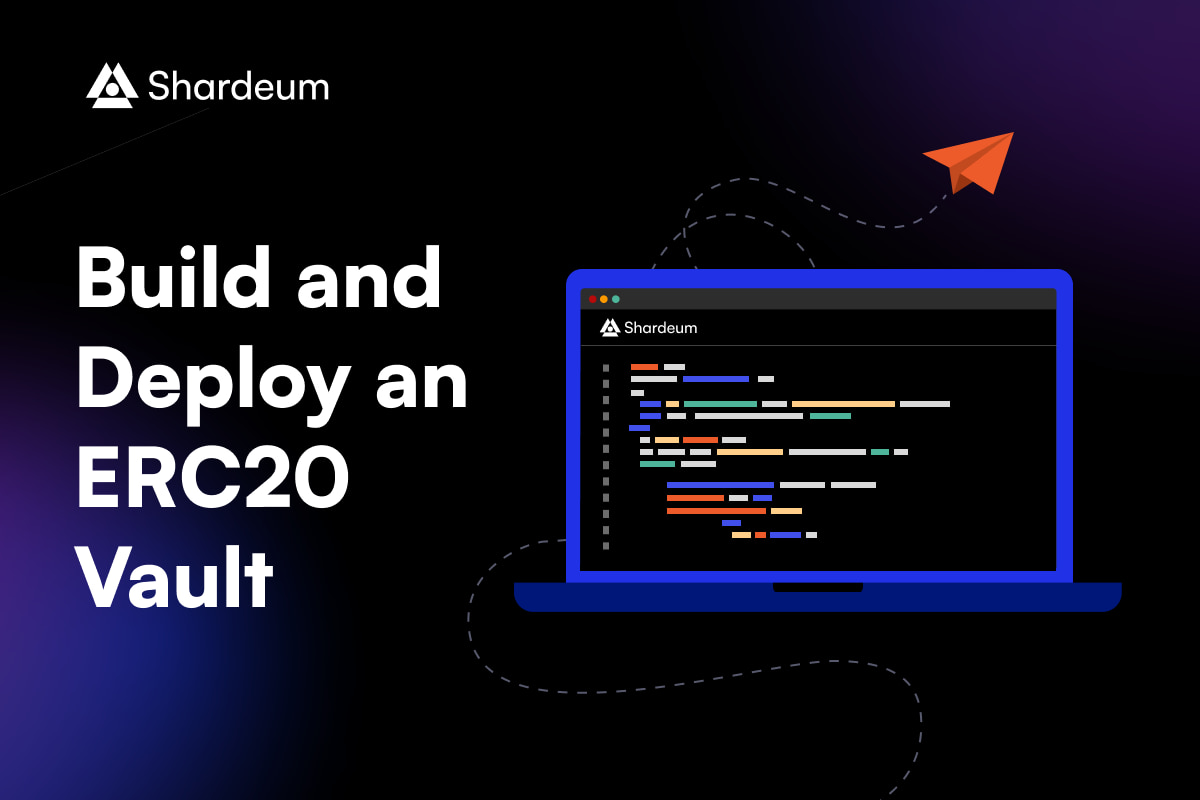
Build and Deploy an ERC20 Vault on Shardeum
In this guide, we are going to learn how to build and deploy a customized decentralized vault smart contract on...
In this guide, we are going to learn how to build and deploy a customized decentralized vault smart contract on...
In this guide, we are going to learn how to build and deploy a customized decentralized vault smart contract on Shardeum. A Vault is a DeFi product where users can stake their tokens. The vault then implements various lending strategies to make a profit and then distribute the profits amongst all the depositors. (Side note: Don’t mistake the topic for Crypto Vault which is a type of a wallet)
For this tutorial, we are going to be using Remix IDE, a popular browser based Solidity IDE.
The basic strategy behind the vault is that when a user deposits ERC20 tokens into the Vault smart contract, we will mint shares (this represents the ownership of respective tokens this user has deposited into the vault contract) and when a user withdraws a token, we will burn the respective number of shares.
With the strategy in place, let’s start with the basic structure of the smart contract.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
import "./IERC20.sol";
contract Vault{
IERC20 public immutable token;
uint public totalSupply;
mapping(address => uint) public balanceOf;
constructor(address _token){
token = IERC20(_token);
}
}
In the above code, we are importing the IERC20 (Interface for ERC20 token which provides us the functions and events needed for the ERC20 token standard).
function _mint(address _to,uint _amount) private{
totalSupply += _amount;
balanceOf[_to] += _amount;
}
function _burn(address from,uint _amount) private{
totalSupply -= _amount;
balanceOf[from] -= _amount;
}
function deposit(uint _amount) external {
uint shares;
if(totalSupply== 0){
shares = _amount;
}
else{
shares = _amount*totalSupply /token.balanceOf(address(this));
}
_mint(msg.sender, shares);
token.transferFrom(msg.sender,address(this), _amount);
}
function withdraw(uint _shares) external {
uint amount = (_shares*token.balanceOf(address(this)))/ totalSupply;
_burn(msg.sender, _shares);
token.transfer(msg.sender,amount);
}
Now, let’s create a standard ERC20 token with the name of “VAULT” and token symbol “VLT” which we will use to deposit in our vault.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
import "./IERC20.sol";
contract ERC20 is IERC20 {
uint public totalSupply;
mapping(address => uint) public balanceOf;
mapping(address => mapping(address => uint)) public allowance;
string public name = "VAULT";
string public symbol = "VLT";
uint8 public decimals = 18;
function transfer(address recipient, uint amount) external returns (bool) {
balanceOf[msg.sender] -= amount;
balanceOf[recipient] += amount;
emit Transfer(msg.sender, recipient, amount);
return true;
}
function approve(address spender, uint amount) external returns (bool) {
allowance[msg.sender][spender] = amount;
emit Approval(msg.sender, spender, amount);
return true;
}
function transferFrom(
address sender,
address recipient,
uint amount
) external returns (bool) {
allowance[sender][msg.sender] -= amount;
balanceOf[sender] -= amount;
balanceOf[recipient] += amount;
emit Transfer(sender, recipient, amount);
return true;
}
function mint(uint amount) external {
balanceOf[msg.sender] += amount;
totalSupply += amount;
emit Transfer(address(0), msg.sender, amount);
}
function burn(uint amount) external {
balanceOf[msg.sender] -= amount;
totalSupply -= amount;
emit Transfer(msg.sender, address(0), amount);
}
}
Now that we have all the smart contracts, let’s start deploying these on Shardeum testnet.
Now, it’s time to test the practical performance of what we just built.
For this example, you can send 100 tokens directly to the Vault contract, assuming that the Vault made some profit.
That’s it!. We just created a vault, then deposited 100 tokens, and eventually withdrew 200 tokens with the profit assumption.
This was a very abstracted and high level implementation of DeFi Vaults. In actual products, there are a lot more factors that will be baked with an optimal business logic to help create profit using various trading/lending strategies.
About the author : Sandipan Kundu is the Developer Relations Engineer at Shardeum. He has been an early contributor to the Web3 ecosystem since 2017 and has also contributed in growing the Polygon devrel team previously.
Building out strong developer evangelism programs with the help of hackathons, workshops, technical content etc to grow and spread the word around for web3 and decentralization has been his primary focus.
Social Links of author :
E-mail : [email protected]
Twitter: https://twitter.com/SandipanKundu42
DAO Guide | Blockchain Beyond Crypto | What is Chainlink | What are Cryptopunks | Advantages and Disadvantages of Decentralization | Peer to Peer Transaction | Web3 Training | Blockchain Proof of Work Vs Proof of Stake | What is Defi 2.0 | What is Phishing and How to Prevent it | Main Features of Web 3.0 | Blockchain Layers Explained | Cryptocurrency Liquidity Provider | EVM Wallet Address | EVM Blockchains Add EVM Network | Custodial Wallets Vs Non Custodial Wallets | Decentralized Identifiers | Cryptocurrency Career Opportunities | What is Consortium Blockchain | Major Components of Blockchain